I want to talk about a common problem that you could face when you develop for Experience Editor.
Imagine that you build your module and it requires Datasource attached to the rendering. If you didn´t add any validation in the view just for the experience editor. Content authors probably experience some errors or couldn’t see the module like the following example:
This is a simple controller which generate a model based on RenderingContext.Current.Rendering.Item
.
public class HeadlineAndDescriptionController : Controller
{
private readonly IViewPathResolver _viewPathResolver;
private readonly IModelMapper _modelMapper;
public HeadlineAndDescriptionController(IViewPathResolver viewPathResolver, IModelMapper modelMapper)
{
this._viewPathResolver = viewPathResolver;
this._modelMapper = modelMapper;
}
public ActionResult Index()
{
var model = _modelMapper.MapItemToNew<HeadlineAndDescription>(RenderingContext.Current.Rendering.Item);
return View(this._viewPathResolver.ResolveViewPath(RenderingContext.Current.Rendering.RenderingItem), model);
}
}
We are going to add the Rendering on a Sample Page, noticed that the Data Source is empty.
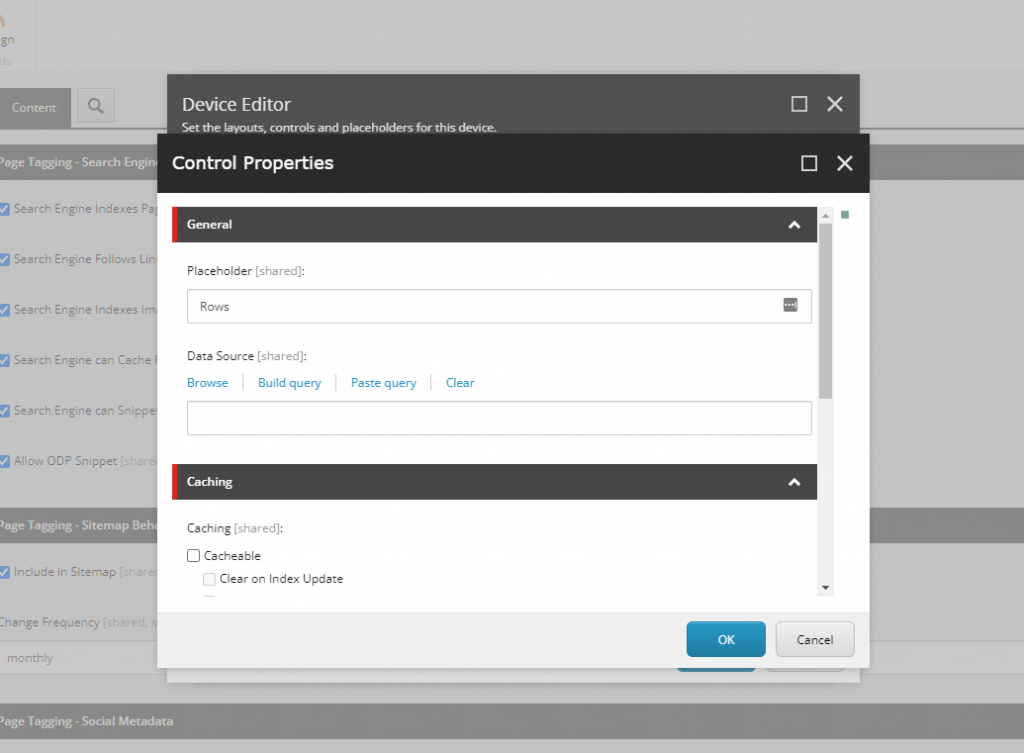
Then if we open the Experience Editor, it generates a bad experience for the content author because we coudn´t find anything on the view in order to associate the desired data source.
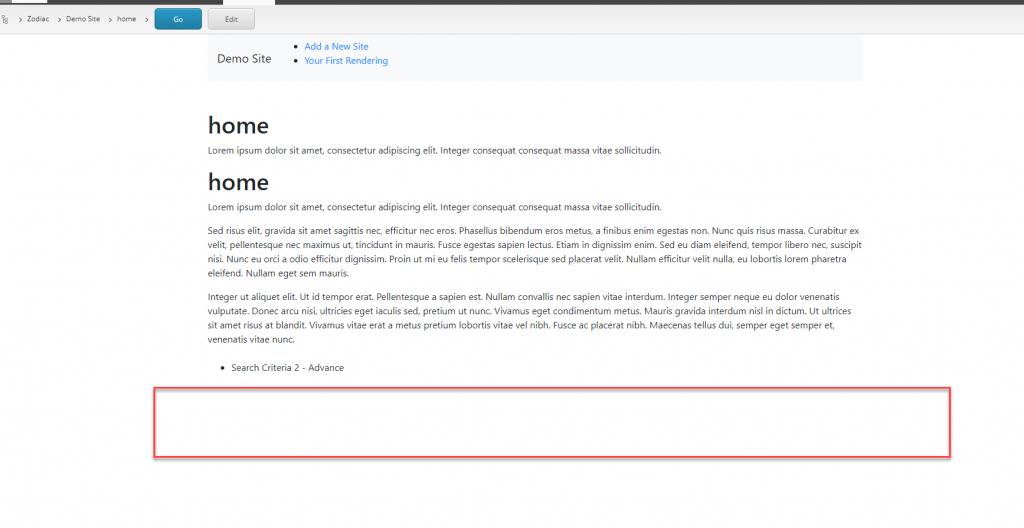
Solution:
In your controller you can add [RequireDatasource]
filter.
[RequireDatasource]
public ActionResult Index()
{
var model = _modelMapper.MapItemToNew<HeadlineAndDescription>(RenderingContext.Current.Rendering.Item);
return View(this._viewPathResolver.ResolveViewPath(RenderingContext.Current.Rendering.RenderingItem), model);
}
This is a simple filter but makes difference for Content Authors:
public class RequireDatasource : ActionFilterAttribute
{
public override void OnActionExecuting(ActionExecutingContext filterContext)
{
if (RenderingContext.CurrentOrNull != null &&
(string.IsNullOrEmpty(RenderingContext.Current.Rendering.DataSource) || RenderingContext.Current.Rendering.Item == null))
{
// Check Experience Editor
if (Context.PageMode.IsExperienceEditorEditing)
{
filterContext.Result = new ContentResult() { Content = @"<p class=""rmc-select-datasource"">[Module: " + RenderingContext.Current.Rendering.RenderingItem.Name + " ("+ filterContext.ActionDescriptor.ActionName+ "): No Datasource Found, Please select Datasource Item]</p>", ContentType = "text/html" };
}
else
{
filterContext.Result = new EmptyResult();
}
}
}
}
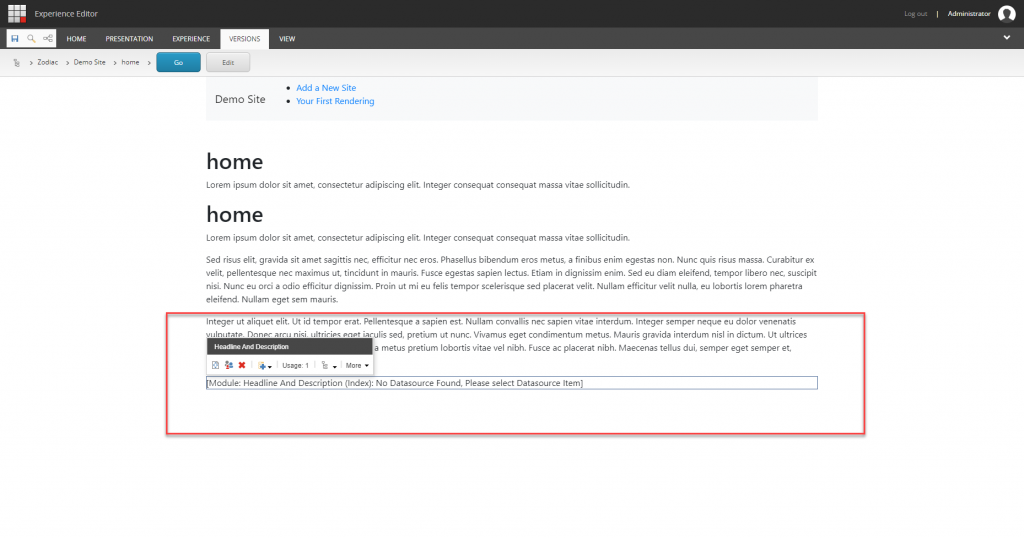
Finally, if you observe a module with message : “Module: {ModuleName} (Method) : No Datasource found, Please select Datasource item”. And content authors know that they need to add a Data source.
I hope this will be helpful to improve the experience for your Content Authors while they are editing.
2 replies on “Sitecore Experience Editor Tips! – Part 1”
Does this also work if a datasource is specified, but someone has unpublished/not published the datasource item? And does it work if using Glass in the controller rendering? (I’d expect that’s a yes.)
Yes!, it does. It works with Glass Mapper also. It validates the rendering item. If you have some logic that abstracts the rendering for you can add the filter at the class definition level.